WelcomeBasicsPlots and GUIApplicationsOther
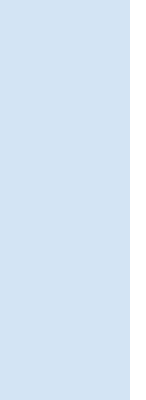 |
Matlab
Examples - matrix manipulation
|
In
this article we study and experiment with matrix manipulation
and boolean algebra.
These Matlab examples create some simple matrices and then
combine
them to form new ones of higher dimensions. We also extract data
from certain rows or columns to form matrices of lower
dimensions.
Let's follow these Matlab examples of commands or functions in
a script.
|
Example:
a = [1 2; 3 4]
b = [5 6
7 9]
c = [-1 -5; -3 9]
d = [-5 0 4; 0 -10 3]
e = [8 6 4
3 1 8
9 8 1]
Note that a new row can be defined with a semicolon ';' as in a, c or
d, or with actual new rows, as in b or e.
You can see that Matlab arranges and formats the data as follows:
a =
1 2
3 4
b =
5 6
7 9
c =
-1 -5
-3 9
d =
-5
0 4
0
-10 3
e =
8
6 4
3
1 8
9
8 1
Now, we are going to test some properties relative to the
boolean algebra...
We
can use the double equal sign '==' to test if some numbers are the
same. If they are, Matlab answers with a '1' (true); if they are not
the same, Matlab answers with a '0' (false). See these
interactive examples:
Is matrix addition commutative?
a + b == b + a
Matlab compares all of the elements and answers:
ans =
1 1
1 1
Yes, all of the elements in a+b are the same than the elements in b+a.
Is matrix addition associative?
(a + b) + c == a + (b+c)
Matlab compares all of the elements and answers:
ans =
1 1
1 1
Yes, all all of the elements in (a + b) + c are the same than the
elements in a + (b+c).
Is multiplication with a matrix distributive?
a*(b+c) == a*b + a*c
ans =
1 1
1 1
Yes, indeed. Obviously, the matrices have to have appropriate
dimensions, otherwise the operations are not possible.
Are matrix products commutative?
a*d == d*a
No, not in general.
a*d =
-5
-20 10
-15
-40 24
d*a ... is not possible, since dimensions are not appropriate
for a multiplication between these matrices, and Matlab launches an
error:
??? Error using
==> mtimes
Inner matrix
dimensions must agree.
Now, we combine matrices to form new ones:
g = [a b c]
h = [c' a' b']'
Matlab produces:
g =
1
2
5
6 -1 -5
3
4
7
9
-3 9
h =
-1 -5
-3 9
1 2
3 4
5 6
7 9
We extract all the columns in rows 2 to 4 of matrix h
i = h(2:4, :)
Matlab produces:
i =
-3 9
1 2
3 4
We extract all the elements of row 2 in g (like this g(2, :)),
transpose them (with an apostrophe, like this g(2, :)') and join them
to what we already have in h. As an example, we put all the elements
again in h to
increase its size:
h = [h g(2, :)']
Matlab produces:
h =
-1
-5 3
-3
9 4
1
2 7
3
4 9
5
6 -3
7
9 9
Extract columns 2 and 3 from rows 3 to 6.
j = [h(3:6, 2:3)]
And Matlab produces:
j =
2 7
4 9
6 -3
9 9
So far so good?
Try it on your own!
From
'Matlab Examples 2' to
home
From 'Matlab
Examples 2' to Examples Menu

|
|
|