WelcomeBasicsPlots and GUIApplicationsOther
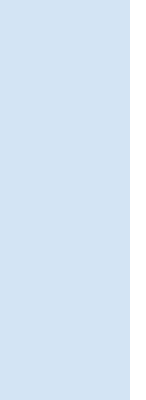 |
Generic
Programming
- Matlab
Cookbook I -
This section includes several examples of generic programming.
These
programs perform a variety of common, practical tasks.
|
Remarks or
comments
are included in the examples to help Matlab learners understand how
each program works.
Roots
of
Quadratic Equations
We
are
going to create now a Matlab program that calculates the quadratic roots (roots of a quadratic
equation). The
equation must be in the following form: ax2
+ bx + c = 0, where a, b, and c are real
coefficients... |
Polygon
Area
This program calculates the area
of a polygon, using Matlab . You must supply
the x and y coordinates of
all vertices. Coordinates must be entered in order of successive
vertices...
Trigonometry
(finding parts of a triangle)
This program calculates three unknown parts of a triangle when three
parts are given (basic trigonometry is used). At least one part given
must be the length of a side.
As in a generic programming way to do it, we show in this code four
possibilities for data entry...
Matrix
decomposition
Matlab includes several functions for matrix decomposition
or factorization.
LU, QR, Cholesky, and SVD built-in functions are explored...
Geometric
Mean and Geometric deviation
This program computes the geometric
mean and geometric
deviation of a set of data. We obtain the results in two
ways, using iterations
and available vectorized
operations in...
Interpolation
This page shows
the most usual and common interpolation concept, as if we were using
generic programming techniques (Matlab has its own function for this
purpose). This code
calculates the y-coordinates of
points on a line given their x-coordinates. It is
necessary to know coordinates of two points on the same line...
Curvilinear
(Lagrange) interpolation
An improvement over the linear interpolation...
Linear
Regression
This program fits a straight line to a given set of coordinates using
the method of least
squares (linear regression). The coefficients of the line,
coefficient
of determination...
Numerical
approximation of a Derivative
We are going to develop a Matlab function to calculate the numerical derivative
of any unidimensional
scalar function fun(x)
at a point x0.
The function is going to have the following usage: D =
Derivative(fun, x0) ...
Numerical
approximation of a Gradient
We are going to include the concepts in our Derivative function created
before, to develop a Matlab function to calculate the gradient of a multidimensional scalar function.
The function is going to have the following usage: g =
Grad(fun, x0)...
Integration
- Trapezoidal Rule
This code approximates the definite integral of a function. The
integral is calculated using the trapezoidal
rule. Parameters of the function are the limits of integration
and the number of intervals
within the limits...
Integration
- Simpson's Rule
This code approximates the definite
integral of a function. The integral is calculated using Simpsons rule. You
must supply the limits
of integration, the increment
between points within the limits, and the function of the curve
to be integrated...
Prime
Numbers
It is well known that prime
factors
of a positive integer are the prime numbers that divide into that
integer exactly, without leaving a remainder. The process of finding
these numbers is called integer
factorization, or prime
factorization. Here’s the full Matlab script...
Euclidean
Algorithm
This
program calculates the Greatest
Common Denominator (GCD) of two integers (see flow-chart).
It is
based on the Euclidean
algorithm for finding the GCD...
Coordinate
Conversion
As another example of generic programming, this couple of Matlab
functions convert the coordinates
of a point given in Cartesian
coordinates to Polar
coordinates, and vice versa. When we use the polar-to-cartesian
function, we enter a magnitude
and an angle in degrees
as parameters. The function returns a real number (x) and a complex number (y value)...
From
'Generic
Programming' to home


|
|
|